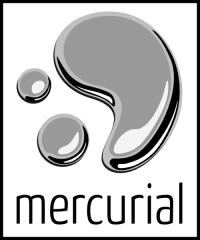
In search for smallest possible Mercurial installation I remembered my old friend FreeNAS. This is small NAS server (at least in 0.7 version) based on FreeBSD 7.3. System requirements are low: 256 MB of RAM and 512 MB system disk is all that is needed. And, of course, you need to have full installation of FreeNAS. Embedded will not suffice.
I will not explain how to setup FreeNAS here since it works out of box with pure defaults. Only thing to configure is where you want your data to be and this should be easy enough to do by yourself (hint: check “Disks” menu). My final goal is to have equivalent for Mercurial under Ubuntu.
First step is to install needed packets. This can be done from web interface (System->Packages) and following packets are needed (given in order of installation):
Now we need to copy some basic files: [bash highlight=“1,2”] $ cp /usr/local/share/doc/mercurial/www/hgweb.cgi /mnt/data/hg/hgweb.cgi $ cp /var/etc/lighttpd.conf /var/etc/lighttpd2.conf [/bash]
We also need a new config file that should be situated at “/mnt/data/hg/hgweb.config” and it should have following lines:
[collections]
/mnt/data/hg/ = /mnt/data/hg/
[web]
baseurl=/hg/
allow_push=*
push_ssl=false
We need to edit “/mnt/data/hg/hgweb.cgi” in order to fix config parameter. Just change example config line with:
config = "/mnt/data/hgweb.config"
At the BOTTOM of existing “/var/etc/lighttpd2.conf” (notice that we created this file via cp few steps ago) we need to add:
server.modules += ( "mod_rewrite" )
url.rewrite-once = (
"^/hg([/?].*)?$" => "/hgweb.cgi/$1"
)
$HTTP["url"] =~ "^/hgweb.cgi([/?].*)?$" {
server.document-root = "/mnt/data/hg/"
cgi.assign = ( ".cgi" => "/usr/local/bin/python" )
}
$HTTP["querystring"] =~ "cmd=unbundle" {
auth.require = (
"" => (
"method" => "basic",
"realm" => "Mercurial",
"require" => "valid-user"
)
)
auth.backend = "htpasswd"
auth.backend.htpasswd.userfile = "/mnt/data/hg/.htpasswd"
}
This config will ensure that everybody can pull repositories while only users in .htpasswd file can push. If you want authorization for pull also, just delete two highlighted files. Notice that users are defined in .htpasswd file that needs to be created with htpasswd command-line tool. Since that tool is not available in FreeNAS easiest way to get user lines is to use online tool.
In order to test how everything works, just go to shell and restart lighttpd with new config:
kill -9 `ps auxw | grep lighttpd | grep -v grep | awk '{print $2}'`
/usr/local/sbin/lighttpd -f /var/etc/lighttpd2.conf start
If everything goes fine, you should be able to access repositories at “http://192.168.1.250/hg/” (or whatever you machine ip/host name is).
As last step we need to add those two commands above (kill and lighttpd) to System->Advanced->Command scripts. Both lines get into their own PostInit command. This ensures that, after every reboot, we start lighttpd with our “enhanced” config file.
P.S. Do not even try to edit “/var/etc/lighttpd.conf”. It gets overwritten after every restart.
P.P.S. This post is just about enabling Mercurial under FreeNAS and because of this it is simplified. It is mere starting point. For further reading check official Publishing Repositories with hgwebdir.cgi guide and my guide on setting-up Mercurial under Ubuntu.