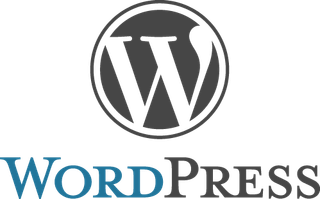
Every WordPress installation is a unique creature as far as customization goes. Mine starts with latest WordPress installation combined with a great free theme called Suffusion. Beauty is, of course, in the eye of the beholder but I believe to be one of best themes out there. It is extremely configurable and you can find it works as well on multimedia-rich sites as on simple home pages as this.
As on all WordPress sites, plugins are plentiful and they change with seasons. Here is my list and reason for them:
Akismet
This is part of probably every blog out there. It will not catch every spam but it will help you deal with most annoying ones out there.
Broken Link Checker
Linking to other websites is path toward hell as far as I am concerned. While it seems as a good idea at first, it can lead to a lot of broken links years down the road. Sometimes nothing can be done about it - resource is simply gone - but this plugin at least makes you aware of it.
CloudFlare
If you use CloudFlare and you love statistics, you will want to install this plugin too. Without it every user would seem to come from the same set of proxied IP addresses and all that per-country log analysis would be for nothing. :)
Facebook Open Graph Meta Tags for WordPress
Simple plugin that does its job - gets your website sharing links for Facebook and Google+ have proper excepts and look decent. It is not fancy and there aren’t many things you can change but I find myself liking all defaults anyhow. I was using NGFB Open Graph+ before but with time it became annoying dealing with its advertisements for bigger Pro edition. Not only that you got a huge banner on your admin pages (sacrilege!) but they started removing features with newer editions (bait, hook & switch). I won’t use it again any time soon.
Fast Secure Contact Form
Simple solution for contact forms. It was a bit annoying to configure, but it worked flawlessly since.
Google XML Sitemaps
Google web crawler occasionally might need a bit of hint as what page is considered more important in your view. 99% of time everything will work properly regardless, this is just to cover all bases.
Limit Login Attempts
Protecting against brute-force password cracking is probably something that should be already built-in to WordPress. But this simple plugin will do to. Security must-have.
Nonsingular noindex
This is a custom plugin I built to avoid Google indexing search and category pages. As blog grew, it became annoying to see search pages in Google results higher than actual page and I had to do something about it. Since I haven’t found any plugin readily available, I decided to build one.
Online Backup for WordPress
If you love your site, you will backup it. And you will backup it offsite. Mailing it to GMail account is perfect for me and this plugin does it without issues.
Simplest icon link
Just a simple plugin to add Apple touch icon to website. Probably there is dozen other plugins that do the same, but I decided to roll my own.
Snippet pre
Never finished plugin for source code highlighting. Since I found every syntax highlighter lacking in some way I decided to build one for myself. While I do use it for new posts, it has severely limited capabilities in its current form.
Snippet text template
One more itch I had to scratch was repeating of same phrases over and over again on multiple pages. So I built this plugin to help me with that. Unsuitable for anybody else because of hardcoding, but it does its job here.
SyntaxHighlighter Evolved
Syntax highlighter that I stopped using because of some annoying bugs and lack of development. However, lot of older posts use it so it will stay here a while. Ultimate goal is to change all those posts to use my own highlighter (once I finish it) but lack of time will probably ensure that never happens.
W3 Total Cache
Probably best caching program out there. If you are using shared hosting and there is any significant traffic, you need something like that. Lot of small options help optimize for your particular situation.
Widget Logic
If you want to limit widgets to some pages only, this is plugin for you.
WordPress HTTPS
This plugin ensures that your login always goes over HTTPS instead of HTTP. Must have if you occassionaly use unknown WiFi to access your blog. Of course, you do need SSL certificate too.