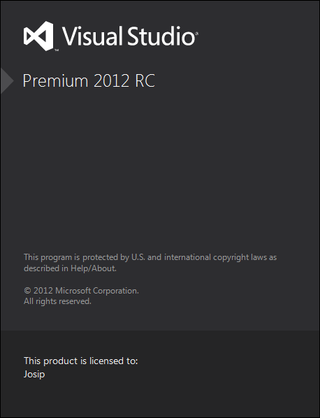
First thing that you will notice when starting Visual Studio 2012 RC is that things got slower. It is not as bad as Eclipse but it is definitely not as speedy as Visual Studio 2010. Second thing you will notice is SCREAMING CAPITAL LETTERS in menu. This change was done in desire to Metro style everything and increase visibility. I can confirm success since it sticks out like a sore thumb.
Fortunately, once you get into editor, everything gets better. There are no major changes for users for old Visual Studio but evolution steps are visible. Find/Replace got major overhaul. Coloring is improved a bit. Pinning files is nice touch. That sums most of it. It was best editor and it remained as such.
Solution window now shows preview when you go through your files so just browsing around saves you a click or two. Beneath each item there is small class explorer. While this might works for small project, I have doubts that it will be usable on anything larger. But I give it a green light since it stays out of your way.
Beautiful change is that every new project automaticaly gets compiled for Any CPU. This is more than welcome change especialy considering utter stupidity from Visual Studio 2010. I just hope that Microsoft keeps it this way. Whoever is incapable of 64-bit programming these days should probably stick to COBOL.
Speaking of utter stupidities, there were rumors that Express editions will not support proper application development but only Metro subset. While I am not against Metro as such, I consider decision to remove Windows application development as good as Windows Phone is. Or, in other words, it might seem logical in head of some manager but in real life there is no chance of this working.
I see Visual Studio Express as a gateway drug toward other, more powerful, editions. Crippling it will just lead to folks staying on perfectly good Visual Studio 2010. Not everybody will have Windows 8 nor care about them. Since I schedule posts few days/weeks in advance, original post had continued rant here. However, there are still some smart guys in Microsoft so desktop application development is still with us in Express.
If I read things correctly it gets even better. It seems that unit testing and source control is now part of Express edition. That were the only two things missing! Now I am all wired up for Express to come. Judging from experience I should probably tone it down unless Microsoft management decides to take some/all stuff away.
All things considered, I am happy with this edition. It is stable, it has no major issues and it is completely compatible with Visual Studio 2010. For most time it feels like Visual Studio 2010 SP2. Try it out.