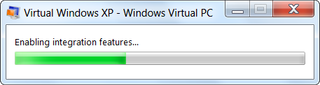
One of optional components of Windows 7 is Windows XP Mode. Idea sounds great: “Let’s make one virtual machine with Windows XP and run all legacy software on it.”. This approach gives you great flexibility in upgrading your software since anything compatible with XP becomes compatible with Windows 7.
In order to use it, additionally to having Windows 7 release candidate, you need to download both beta of Virtual PC 2007 and beta of Windows XP mode.
How does it look
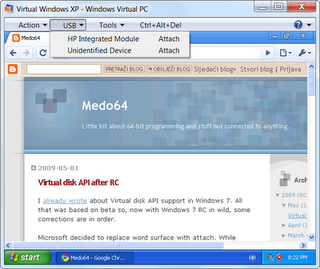
Interface is really minimalistic. I do miss hard disk activity icon in status bar, but if you consider that plenty of people who have no idea what virtualization is will use it, that may not be bad idea. All other options seem to be in place (not that Virtual PC had much of options anyhow).
New Virtual PC
Since this feature is based on new Virtual PC, this is great chance to see how things will look with new version.
First thing that I noticed is USB menu. Believe it or not, it seems that Microsoft finally decided to add some support for USB devices. I tested it with USB drive and it worked (after automatic installation of small stub driver), but I cannot vouch for more complicated devices. For some devices (in my case VGA camera) it shows unidentified device, but attaching it does work properly so I will give it benefit of doubt and assume that this is something inherit to beta or my laptop.
Everything else seems to be same like in Virtual PC 2007 but with less flexibility (no separate virtual disk wizard, no device activity icons…). Hopefully advanced interface (like one in old Virtual PC) will be available after this beta.
Auto publishing
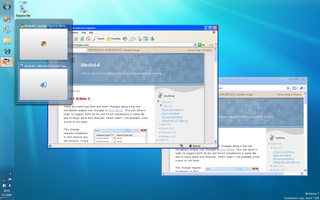
When I saw picture of Windows XP mode in action for the first time, I liked feature that they called auto publishing (to me it looks same as VirtualBox’s seamless mode).
In theory it seemed simple, you just install some legacy application in Windows XP (for me it was Google’s Chrome - it still doesn’t work on Windows 7 - shame on you Google) and auto publishing should add shortcut to that application in Windows 7 menu. Once you start your application via that shortcut, you get desktop integration and all that stuff.
Problem with that simple scenario is that it does not work. In moment of pure desperation, I even tried looking it up in help. That action wasn’t helpful since documentation is still not done and all I got was equivalent of “This page was intentionally left blank”. By pure accident, I stumbled upon Virtual PC forum. Only there I saw how that should be done. You need to manually create “Programs on the XP VM” folder under “C:\Documents and Settings\All Users\Start Menu”. Once shortcut gets placed there, it also gets copied to Windows 7 menu.
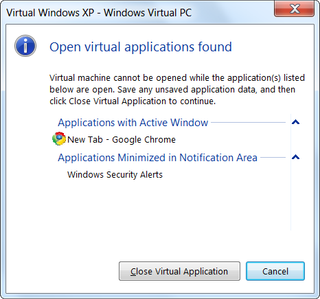
Another thing that can cause some problems is fact that you cannot both see desktop and applications at same time. Although this is not a big problem in normal circumstances, it causes minor inconvenience when you need to go in Control Panel (or some other non-published application) since you need to save your work first. Same thing will also happen if you go from full desktop to application mode.
There are minor issues with Windows 7 specific features and application virtualization. One that I mind most is missing thumbnail preview on taskbar button. If you have few applications open (e.g. few instances of Visual Basic 6.0), it is impossible to tell them apart without actually switching.
Other minor issues
For this to have any chance of working, you need hardware support for virtualization. I do not quite get why this is prerequisite. Everything that you could do with hardware supported virtualization, you could do without it also (with some speed loss). [2010-03-19: Hardware supported virtualization is not needed any more.]
Speaking of speed, starting time for this is not so good. It takes a while for this virtual machine to boot. While I do understand reason behind slow start, I am not so sure that someone using it on daily basis would be happy with that explanation.
I also doubt decision to make hibernate a default for close action. Hibernate may seem like a decent option at first, but probability is that some imaginary “default” user will probably always perform close (and implicitly hibernate it every time). I am not that sure that application that hasn’t fix it’s compatibility bugs for last seven years will take hibernate kindly. Expecting inexperienced user to find shut-down option behind Ctrl+Alt+Del and know when to do it is little too much of optimism for my taste.
Is it any good?
For those clients stuck with some legacy application, I would say yes. You get great new interface and features, along compatibility with your Clipper-like weight. For programmers - definitely - you get one free XP system to test your programs on.
All others can safely ignore it.