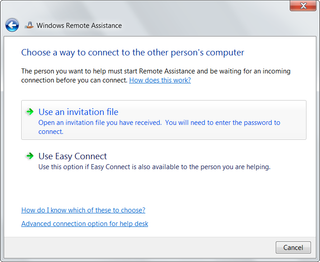
I needed to do some programming on other PC and Remote Desktop was obvious solution. However, since it wasn’t my computer, I felt uneasy doing something while other person could see it. Next best choice was Remote Assistance.
If you intend to write code via Remote Assistance be aware of some issues:
1. Mailing invitation doesn’t work every time.
For some reason, mail invitation works in aproximatelly 50% of cases. I could blame corporate firewalls and proxies, but it seems to me that, if you are going to have such feature, you need to anticipate those problems.
2. You need to work in window.
For some reason, you cannot go into full screen in order to see other side. You will stay in window and, since there is chat window and some status around, destination needs to be a lot smaller. My home resolution was 1280x1024 and I could manage only 800x600 on other side. Second solution would be to scale window, but it is very hard to program if all you see is bunch of pixels.
3. Escape key is used for disconnect
Every time you hit escape key, you will disconnect remote control mode. In order to continue working other side will need to approve you again. And again. And again. If you are used to close windows with escape key (e.g. Intellisense windows), you will disconnect a lot.
Reasoning behind that “feature” was understandable. Allow user to disconnect at any time if he/she sees suspicious behaviour of repair-man. However, that does not explain why it disconnects when remote side hits escape button also. I doubt that there is scenario when remote side wants quick way out - especially mapped to escape key.
I did solve it after ten or more disconnects. I took screw driver and popped it out.
Conclusion
While I cannot say that I enjoyed this much, it was enough to get job done. I assume that with this solution it is as good as it will get.