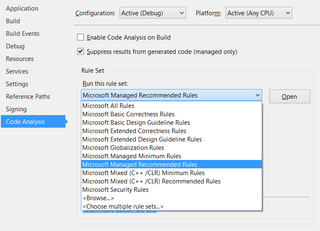
Static code analysis is a beautiful thing. For a while now Visual Studio has it embedded and it is only a right click away.
By default each project gets assigned “Microsoft Managed Recommended Rules” as baseline for analysis. In my opinion this is really good choice for most of projects. It does include quite a few checks (61 to be exact) and whatever you get is almost certainly something you want to improve.
I would argue that most projects would benefit from setting bar quite a bit higher at “Microsoft Extended Design Guidelines Rules”. This ruleset gets annoying pretty fast when you run it on existing project. You can almost be sure that code will be peppered with CA1704: Identifiers should be spelled correctly
and CA1062: Validate arguments of public methods
.
First one can be sorted with small code analysis dictionary and second one will need few if
s here and there. All other warnings can also be sorted with similar level of effort since they are not real errors nor something that will cause immediate problems (e.g. double dispose). If project is old this can take ages and it is for little or no practical value.
Biggest beast is “Microsoft All Rules” setting. This one will complain for anything and everything and it will be really hard to satisfy it in any GUI project. Yes, it is possible but this purity brings no value to customer and I usually keep my main assemblies away from it.
I have quite a simple personal rules which level to use. If assembly is intended to serve as a framework that will be written in stone once it is released I go with “Microsoft All Rules”. It is annoying at times but users of your framework will be thankful because it forces really tidy external interface.
If assembly is intended for sharing between projects I go with “Microsoft Extended Design Guidelines Rules”. It forces me to keep design guidelines close to mind and to avoid some potential bugs that can just creep in code (e.g. returning internal array directly). All externally visible members should be held to higher standards since you never know when you or someone else might reuse them.
If given assembly contains GUI, I usually just stick to default Microsoft Managed Recommended Rules. GUI assemblies are not intended to be called directly and bunch of warnings will be just annoyance (e.g. localization warnings when localization is not even planned).
Your mileage and opinion might differ but one thing is for sure - any project has to have at least minimum code analysis enabled.
PS: As number of rules increases so will number of false warnings. Suppressing code analysis warnings is sometime only way to go around it. Just make sure you have really good reason. If you have too much (valid) suppressions that might as well mean that you selected wrong code analysis level for your project.
PPS: It is not always clear which rule belongs to which ruleset. Therefore I have prepared small Excel file with this information.