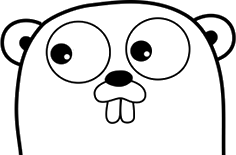
After sticking with C# and its ugly step-mother Java for a while, I though it was a time to check out a new language. One that seemed interesting was Google’s Go, a simple garbage-collected, strongly-typed, and C-like language supporting Linux and Windows.
Go syntax itself is really friendly and suits me well. There are only a couple of statements around and you should know them from C, semicolon is optional at many places, and in-place variable initialization is a treat. Yes, x := 5
is not really superior to var x = 5;
nor it saves you a lot of keystrokes. However, syntactic sugar is what makes or breaks a language in my opinion. And such lazy variable initialization is nice to have.
Cross-compilation is reasonably easy with just a few environment variables (GOARCH
and GOOS
) that need adjusting. As it is statically linked, you can count on having no additional dependencies whatsoever. One binary is all it takes. Yes, binary is a bit bigger even for the simplest of things but I’m perfectly fine with that if I can avoid .dll hell.
Speaking of compilation, it is annoying. Compiler is simply too aggressive with warnings. Great example is if you initialize variable and you don’t use it later. Damn compiler will complain and refuse to do its work. If you have unused import, the same thing. It will simply stop at any warning. One might say this is the correct behavior and that it will help you to write better code. That hypothetical guy should burn in hell next to guy who created this compiler.
Since proper debugging tools for this language are nonexistent, you are pretty much forced to use generous peppering of printf
statements throughout the code together with liberal commenting out so that you can pinpoint the error. And guess what happens as you debug it? Damn compiler refuses to work just because I am no longer needing one variable I used for debugging or because I am importing package use of which is currently commented out.
And those are errors compiler correctly identifies and it could correct itself. Variable not used? Report me a warning because it might be me making a typo but compile the damn program so I can continue to debug. Same for extra package - if you are so smart to report it is unused, remove the damn thing yourself and compile. It is impossible to describe how annoying these warning are during writing and debugging of program. ANNOYING.
Syntax of Go is mostly pleasant with a couple of weird decisions most notable of which is to have variable type after the variable name, as in func x(dummy int)
. While designers do offer an explanation, to me frankly it seems as changing stuff just to be different. Yes, it might be more correct way of doing things but it goes against muscle memory of every developer on earth. Same goes for decision to use nil
instead of null
. Why?
Go is not object-oriented language and I am not really sure how I feel about it. A wonders can be done without full OO support, especially when it comes to small tools I was using it for. What I was missing were two features usually connected to OO languages.
First one is syntactic sugar of method calling syntax. I find myStr.TrimSpace()
as superior compared to strings.TrimSpace(myStr)
. While they can both serve the same function, I find former much easier to both write and read. I sort-of expected Go to have something similar to C# extension methods where you essentially just use OO syntax for non-OO concept.
Second is method overloading. Yes, I know Go creators have excuse for this too. Who knows, they might even be technically correct. However, the need to have slightly different name for each method taking similar parameters is annoying. Have they allowed optional parameters maybe I would feel different about it but, as it is implemented now, I find this decision hurting the language.
Lastly among complaints is lack of globalization features throughout the language. It could be at least partially due to the lack of overloading but all globalization features feel as an afterthought and not as the part of language. Good luck localizing this.
As you might have guessed, I don’t find Go a particularly well designed language. I do like some of its features (especially the ease of cross-compiling) but general discomfort during development will keep it as tool of choice only when I want a single binary with minimal impact to the rest of system and not for much else.